The latest version of the Kundalini Piano Mirror supports Computer Assisted Dynamics!
An embedded metronome serves the same purpose as a traditional metronome, but has the added benefit of allowing the Piano Mirroring system to know which measure of a piece a performer is on.
Additionally, the system now supports user-supplied .Lua scripts, which execute in real-time; this is possible because the Lua interpreter is now compiled into the Piano Mirror.
Lua is a powerful, efficient, lightweight, embeddable scripting language, […], making it ideal for configuration, scripting, and rapid prototyping.
https://www.lua.org/about.html
By writing a .Lua script for each passage one is working on, it makes it possible for the Piano Mirror system to help the pianist achieve the sound they are looking for.
One way to conceptualize of this is to think of it as sort of like “training wheels” or “self-driving mode” for your piano: YOU still have to drive (play), but the piano KNOWS what you are TRYING TO DO, and helps you achieve it.
As a simple example, imagine the following coda from the Schubert Impromptu in F Minor, Op. 142 no. 4:
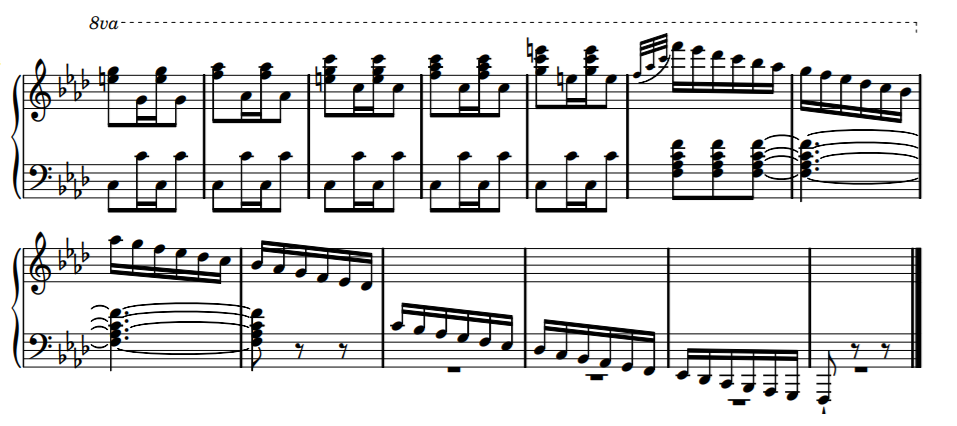
As can be seen, the piece ends with a 7 octave descending F minor scale.
One possible interpretation is to play the entire scale using a decrescendo. Assuming this is our intention, a script similar to the one below could be developed:
--[[ This script is designed to illustrate a 7 octave decrescendo, as is found in the code of the Schubert F Minor Impromptu. The idea is to execute a smooth decrescendo across the range. However, just for a better musical effect, the rate of the decrescendo is increased several times during the run. ]] --[[ function process_midi() is called by the Kundalini Piano Mirror any time a MIDI event is received. So in here, we can do anything we want to status, data1, and data2... and then just return them before the PianoMirror writes out the data data1 is the MIDI note number data2 is the volume (where 0 means key-up) ]] function process_midi(status, data1, data2) -- if this is a keyup, then don't do anything, just return -- whatever was sent in if (data2 == 0) then return status, data1, data2; end -- start the decrescendo when we the high F; we start by decreasing the volume by 1 unit for each note... if (data1 == 101) then print('point 1...'); volume = 90 -- set initial volume to 90 (= Loud) increment = 1 end --when we get to f two octaves below, start decreasing volume by 2 units each note... if (data1 == 77) then print('point 2...'); increment = 2 end --and finally, for the last octave, reduce really fast... if (data1 == 41) then print('point 3...'); increment = 3 end volume = volume - increment; print(volume) return status, data1, volume; end -- code down here runs when the script is initially loaded... -- so we just need to initialize everything volume = 90 increment = 1 print("example 7 octave decrescendo");
(The script above is heavily commented to serve as an example; normally a script would probably be less verbose.)
The script above is interesting, but is obviously only one example of the type of thing that is possible. (For example, we could just as easily make a script that performs a crescendo instead.)
The way the script works is to help the piano perform a smooth decrescendo across the entire 7 octave range. However, to make things sound even more musical, the rate at which the crescendo occurs increases 2 times — which creates a beautiful musical effect.
(In future posts, I will provide more examples, illustrating how the scripting works, and how to use it to achieve various musical effects such as to facilitate 3-part writing, for example. For now, the take-away should just be that it is possible for the pianist to write a simple script that instructs the Piano Mirror System how to help him achieve pianistic affects.)
Conclusion
The perennial task of a musician consists of the process of continually refining one’s technique so that one can more readily achieve one’s artistic aims.
By allowing a pianist to program the Piano Mirror system to help with dynamics and phrasing, a pianist is able to further bridge the gap between her performed reality and her artistic ideal.
Next Steps
- Stay tuned for more videos and documentation on how to create the scripts